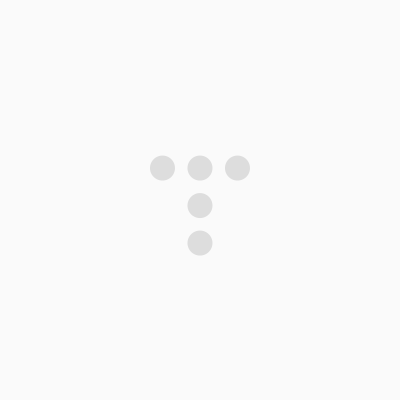
|
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
/* ========================================================================== */
/* */
/* magic_odd.c */
/* 2007.06.20., Naska */
/* */
/* magic square(odd) */
/* */
/* ========================================================================== */
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
/* undefine symbols */
#ifdef ERR
#undef ERR
#endif
#ifdef OK
#undef OK
#endif
/* define symbols */
#define ERR (-1)
#define OK (0)
#define LINE "----"
/* define macro */
#define CORR_POS(x, y) {\ \\바운더리 넘었을지 모르니까 체크를 해주는 것 혹시 0및으로 떨어지거나 맥시멈값이 4인데 4를 넘어서 5이상으로 되지 않나 하는것
x %= size;\
y = y < 0 ? y + size : y % size;\
}
#define CHANGE_NEXT_POS(x, y) {\\다음에 어디에 정보를 채워 넣을 것인지 좌표를 바꺼주는것이다.
int ix = x, iy = y;\
x ++;\
y --;\
CORR_POS(x, y);\
if (cell[y][x] != 0) {\
x = ix;\
y = iy + 1;\
CORR_POS(x, y);\
}\
}
/* functions */
void print_usage(char *prog);
void create_ms_odd(int *malloc_ptr, int size);
void print_ms_odd(int *malloc_ptr, int size);
int main(int argc, char *argv[]) //메인의 아규먼트로 칸수를 받는다.
{
int cell_size;
int *cell_array;
if (argc != 2) { //사용법을 찍고 넘어온다.
print_usage(argv[0]);
return (ERR);
}
cell_size = atoi(argv[1]); //아스키 문자열을 인트져형의 숫자로 바꺼준다.
if (!(cell_size % 2)) { //짝수면 에러
printf("cell size must be odd\n");
return (ERR);
}
printf("Cell size : %d\n\n", cell_size);
cell_array = malloc(cell_size * cell_size * sizeof(int)); //하나만써서 전체크기를 우선 할당받음 이걸 보면 1차원 배열밖에 안된다.
if (cell_array == NULL) { //널이면할당이 안되서 에러메서지
printf("memory allocation error!\n");
return (ERR);
}
memset(cell_array, 0x00, cell_size * cell_size * sizeof(int));
create_ms_odd(cell_array, cell_size);//할당받은 포인터를 넘기고 셀사이즈를 넘긴다.
print_ms_odd(cell_array, cell_size);
return (OK);
}
void print_ms_odd(int *malloc_ptr, int size)
{
typedef int (*cell_t)[size];
cell_t cell;
int sum[2][size];
int x, y;
cell = (cell_t)malloc_ptr;
memset(sum, 0x00, sizeof(sum));
for (y = 0; y < size; y ++) {
for (x = 0; x < size; x ++) {
printf("%4d", cell[y][x]);
sum[0][y] += cell[y][x];
sum[1][x] += cell[y][x];
}
printf(" |%4d\n", sum[0][y]);
}
for (x = 0; x < size; x ++) {
printf(LINE);
}
printf("\n");
for (x = 0; x < size; x ++) {
printf("%4d", sum[1][x]);
}
printf("\n");
}
/*
void func 2(void* func,int a){
}
void func 2(func_t func,int a){
}
//함수 포인트의 타입typedef int (*func_t)(void);
*/
/* vfs 가상파일 시스템
오픈이라는 함수를 거치면 Vfs가상파일시스템을 거쳐서 뒤에 나와 있는 디바이스에 접근을 하기 시작한다.
open(" ",----);
해당하는 ----장치의 오픈에게 전달인자를 주면
----장치가 오픈을 하고 클로우즈한다.
*/
void create_ms_odd(int *malloc_ptr, int size)
{
//배열포인터 int (*cell)[10]; 1개증가할때 40씩증가
//포인터배열 int *cell[10]; 1개증가할때 4증가
typedef int (*cell_t)[size]; //이렇게 쓰면 타입을 선언해줌 셀언더바 타임이 이런형태의 타입이된다.
cell_t cell; //이타입으로 셀을 선헌
int num, x, y;
cell = (cell_t)malloc_ptr; //셀이라는 변수를 이차원 배열 처럼 쓸수 있다.
x = size / 2;
y = 0;
for (num = 1; num <= size * size; num++) {//1부터사이즈까지 포문을 돌리면서 셀을 채워준다.
cell[y][x] = num;
CHANGE_NEXT_POS(x, y);
}
}
void print_usage(char *prog)
{
printf("usage : %s <cell size(3~17)>\n", prog);
}
sokoban's Blog is powered by Daum & Tattertools