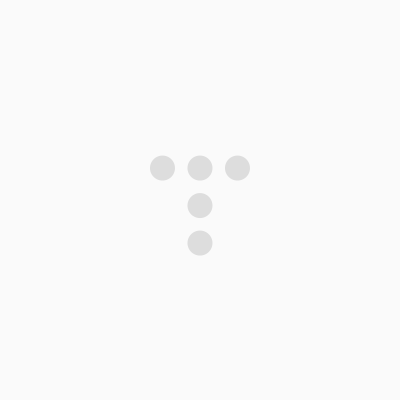
|
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
-
26_5번에서 메세지를 쓰고 대기 하고 있고
26_6번에서 메세지를 읽으면 그값을 출력하고 프로세스를 둘다 종료한다.
--------------------------------------26_5----------------------------------------------------
#include <stdio.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <signal.h>
#define SIZE 1024
void signalHandler(int signo);
int shmid;
main()
{
void *shmaddr;
/* 1234 키의 공유메모리 생성 */
if((shmid=shmget((key_t)1234, SIZE, IPC_CREAT|0666)) == -1) {
perror("shmid failed");
exit(1);
}
/* shmid 공유메모리를 호출 프로세스 메모리 영역으로 첨부 */
if((shmaddr=shmat(shmid, (void *)0, 0)) == (void *)-1) {
perror("shmat failed");
exit(1);
}
/* 공유메모리에 데이터 쓰기 */
strcpy((char *)shmaddr, "Linux Programming");
/* 공유메모리를 호출 프로세스의 메모리 영역에서 분리 */
if(shmdt(shmaddr) == -1) {
perror("shmdt failed");
exit(1);
}
/* SIGINT 시그널 받으면 signalHandler 실행하도록 설정 */
signal(SIGINT, signalHandler);
pause();
}
void signalHandler(int signo)
{
/* shmid 공유메모리 삭제 */
if(shmctl(shmid, IPC_RMID, 0) == -1) {
perror("shmctl failed");
exit(1);
}
exit(0);
}
----------------------------------------26_6----------------------------------------------------
#include <stdio.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <signal.h>
#define SIZE 1024
main()
{
int shmid;
void *shmaddr;
struct shmid_ds shm_stat;
/* 1234 키의 공유메모리 있으면 접근해서 식별자 얻음 */
if((shmid=shmget((key_t)1234, SIZE, IPC_CREAT|0666)) == -1) {
perror("shmid failed");
exit(1);
}
/* shmid 공유메모리를 호출 프로세스 메모리 영역으로 첨부 */
if((shmaddr=shmat(shmid, (void *)0, 0)) == (void *)-1) {
perror("shmat failed");
exit(1);
}
/* 공유메모리에 저장된 데이터 출력 */
printf("data read from shared memory : %s\n", (char *)shmaddr);
/* shmid 공유메모리 정보를 얻어 shm_stat에 저장 */
if(shmctl(shmid, IPC_STAT, &shm_stat) == -1) {
perror("shmctl failed");
exit(1);
}
/* 공유메모리를 호출 프로세스의 메모리 영역에서 분리 */
if(shmdt(shmaddr) == -1) {
perror("shmdt failed");
exit(1);
}
/* shm_stat.shm_cpid 프로세스에게 SIGINT 시그널 보냄 */
kill(shm_stat.shm_cpid, SIGINT);
exit(0);
}
sokoban's Blog is powered by Daum & Tattertools